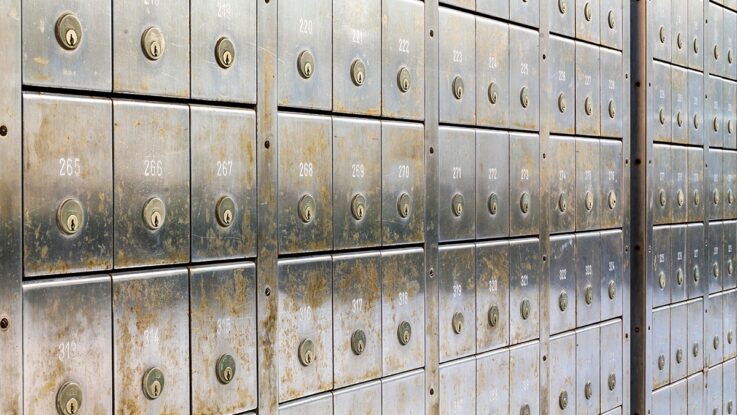
Question: How do I keep my API keys from becoming part of someone else’s GitHub search?
Answer: Storing API keys directly in your code is generally not recommended due to the potential security risks. If your code is ever shared or becomes publicly accessible, anyone who sees the API key can use it, potentially leading to abuse or a security breach.
There are several ways to store API keys securely:
Let’s take a look at how to implement the first two, the lowest-overhead of these solutions, in secure coding. I’ve used Python as the example language for this, although any language will have similar concepts. The advantage of Python is its simplicity and ease of use, which together with its formidable data handling capabilities make it ideal for most uses.
To Use Environment Variables for Storing API Keys
Environment variables allow you to store sensitive information, such as API keys, outside of your source code. They are accessible to the application during runtime and can be easily configured on different environments (e.g., development, staging, and production).
First, set the environment variable in your system or the platform where your application is running. In a Unix-based system, you can set an environment variable using the export command:
Next, access the environment variable in your application’s code. For example, in Python, you can access the value of an environment variable using the os module:
To Use External Configuration Files for Storing API Keys
Another way for the beginning developer or data scientist to store API keys is via external configuration files. These can be very useful; however, you should exclude them from the repository using .gitignore or the equivalent.
First, create a configuration file, such as config.json, and store the API key in it:
Next, add the configuration file to your project’s .gitignore (or equivalent) to ensure it’s not tracked by your version control system.
Finally, load the configuration file in your application’s code to access the API key. For example, in Python, you can load a JSON configuration file and access the API key like this:
Lock Up Your APIs
By using either environment variables or external configuration files, you can better protect your API keys from accidental exposure, simplify management of sensitive information, and make it easier to maintain different configurations for various environments. You should enforce proper access controls on the environment variables and configuration files to prevent unauthorized access.
As your project grows in complexity (and importance), it may be advisable to move to platforms with innate capability such as secrets management systems or environment-specific configuration services for storing sensitive items. However, these quick solutions help you start off on a more secure footing.